I love junior devs. There aren't many places—work, school, anywhere—where you'll find people that hungry to learn, that fired up by what they're building. I remember that feeling. Programming was new. It was exciting. I wasn't just taking what the machine gave me—I was telling it what to do. I didn't always understand why it worked, but it did. The computer listened.
That feeling doesn't last.
Eventually, the magic wears off. Things that used to feel powerful start to feel routine. You don't lose the passion, exactly—it just shifts. The buzz you got from seeing something work gets replaced by deadlines, code reviews, and bug tickets. It becomes a job.
But the ones who stick with it? They figure out how to keep going. They stay curious. They chase the puzzle. They learn how to solve real problems—and they get better because of it.
Still, right after that first high, there's usually a crash. Somewhere between your first finished tutorial and your first real app, the spark sputters. You stall out.
You hit a wall.
Syntax Isn't the Hard Part
Getting to mid-level isn't just about more time behind the keyboard. It's where a lot of people stall out. You've learned the syntax—data types, loops, functions—you know how code works. But no one told you what to do with it.
I remember finishing Learn Python the Hard Way back in 2015. I closed the book, sat in my chair, and thought: What do I even make?
I started digging around for inspiration and found a tutorial for a Twitter retweet bot. It looked cool and seemed easy enough. I followed along and ended up with a bot that retweeted any post with the words "dark souls." (That account still exists, but I don't remember the login.)
And that was it. That's where my Python journey ended. Not because I didn't enjoy it—I just didn't know where to go next. I had no plan. I wanted to learn how to program, and technically I did. But I never figured out what to do with it.
What not to do
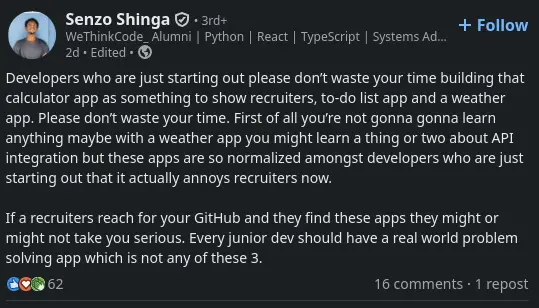
That LinkedIn post kicked off this whole thing—and I agree with it.
Don't showcase tutorial projects.
Unless you're vastly improving on the original idea.Tutorial projects are good introductions to concepts, but they don't prove you know how to program—they prove you can follow instructions. At best. At worst, they show you know how to copy and paste.
Side note: The perfect tutorial doesn't exist. Pick one and move on.
"Ok, so what do I build?"
When I was a TA—and later as a senior dev—I always told junior developers struggling with a concept to make it personal. Tutorials are generic by design. They use bland, abstract examples that aren't tied to anything you care about. But when you use something relevant to you, the concept clicks faster.
Take JavaScript objects. A junior dev runs into trouble, Googles it, lands on MDN. What's the first example they see?
const obj = {
foo: 1,
// You should not define such a method on your own object,
// but you may not be able to prevent it from happening if
// you are receiving the object from external input
propertyIsEnumerable() {
return false;
},
};
obj.propertyIsEnumerable("foo");
// false; unexpected result
Object.prototype.propertyIsEnumerable.call(obj, "foo");
// true; expected result
The MDN docs are an incredible resource—I've referenced them more times than I can count—but this example looks like it was written for a robot. And right out of the gate, it shows you something you shouldn't actually do. If you want to actually understand something, make it personal.
So I'd ask, "What's something you like?"
"Skateboarding."
"Cool. Let's go with that."
const skateboard = {
bearings: "Bones Reds",
deck: "Traffic 8\"",
griptape: "Mob Grip",
hardware: "Ace",
wheels: "Spitfire 53mm",
hasRazortail: function () {
return false;
}
}
console.log(skateboard.wheels);
// "Spitfire 53mm"
console.log(skateboard.hasRazortail());
// false
Adding your own personality helps it stick. So when you're trying to recall what an object is, you're not reaching for some bland MDN example—you're thinking about your skateboard, or whatever it is you care about.
"You still haven't told me what to build."
Exactly. That's the point—build something you care about. Solve a problem in your life, no matter how dumb or minor. Or build something completely ridiculous. It doesn't matter. Just make it yours.
Once, during a company meeting, I came up with this silly idea: Shopping Cart Roulette. In the daydream version, a wheel spins—five slices give you a discount, but the sixth is a bullet. Land on that, and boom: the most expensive item in the store gets added to your cart. (In hindsight, the bullet emptying the cart would've been funnier.)
So I started building. I broke down the logic right away: random number generator, the wheel was just flavor, and the result either applied a coupon or added a product. Easy. Within an hour, I had a working prototype.
But that's when the real ideas started.
“What if the customer could choose what to add?”
“What if they could see everything they recently viewed?”
And that's how I came up with the concept for the Recently Viewed Addon.
Nine times out of ten, you'll start with something small, and it'll snowball into something bigger than you imagined. Programming takes creativity. And the best way to break through a block is to just start building.
If all else fails, rebuild a site you like—but strip out all the junk. Every site is bloated with nonsense. Cutting it down should be the easy part.
Or run drills on the concepts that don't fully click. I used to just type out for
loops over and over because I couldn't quite picture what was happening in my mind's eye. Sometimes you have to brute-force it until it inspiration comes.
At the end of the day, the best way to learn is to build—and keep building.